If you’re a Linux user and you work with images in your web development projects, you might have come across the need to manipulate images — resizing, cropping, converting formats, and more. While there are many libraries out there, one of the most popular and powerful ones is Sharp.js.
In this blog post, we’ll dive into why Sharp.js is the best Node.js image framework, especially for Linux users who are just starting out. We’ll also explore how to install it and use it for basic image operations.
Table of Contents
What is Sharp.js?
Sharp.js is an image processing library for Node.js that provides a high-performance API for manipulating images.
It supports a wide range of operations, including resizing, cropping, rotating, and converting image formats. The framework is built on top of the libvips
library, which is known for its speed and memory efficiency compared to other image processing libraries like ImageMagick or GraphicsMagick.
Sharp.js can handle a variety of image formats, including JPEG, PNG, WebP, TIFF, and GIF, which is particularly well-suited for applications that need to process large numbers of images or require fast processing times.
Installing Sharp.js on Linux
Before you start using Sharp.js, you need to have Node.js installed on your system. If you don’t have it installed, you can install it from our guide: How to Install NodeJS in Linux.
Once Node.js is installed, you can proceed with the installation of Sharp.js as shown.
mkdir sharp-image-processing cd sharp-image-processing npm init -y npm install sharp
After installation, you can verify that Sharp.js is installed correctly by checking the version:
node -e "console.log(require('sharp').version)"
If everything is set up correctly, you should see the version number of Sharp.js printed in the console.
Using Sharp.js for Image Processing
Now that Sharp.js is installed, let’s explore some common image manipulation tasks.
1. Resizing an Image Using Node.js
Resizing images is one of the most common tasks in image processing and sharp.js makes it easy to resize images with just a few lines of code (resize.js
).
const sharp = require('sharp'); sharp('input.png') .resize(300, 200) // Resize to 300px by 200px .toFile('output.png', (err, info) => { if (err) { console.error('Error resizing image:', err); } else { console.log('Image resized successfully:', info); } });
After creating your script, run it using Node.js:
node resize.js
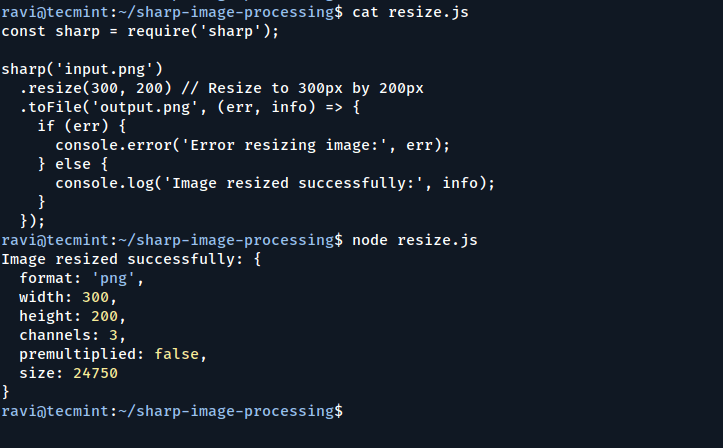
In this example:
- The input image (
input.png
) is resized to 300px width and 200px height. - The output is saved as
output.png
.
You can also maintain the aspect ratio by providing only one dimension (width or height), and Sharp.js will automatically adjust the other dimension.
2. Cropping an Image Using Node.js
Sharp.js allows you to crop an image to a specific region.
sharp('input.png') .extract({ left: 100, top: 100, width: 400, height: 300 }) // Crop the image .toFile('cropped.png', (err, info) => { if (err) { console.error('Error cropping image:', err); } else { console.log('Image cropped successfully:', info); } });
The extract method takes an object with left
, top
, width
, and height
properties, defining the region to crop.
3. Converting Image Formats Using Node.js
Sharp.js allows you to easily convert images between different formats.
sharp('input.png') .toFormat('jpeg') .toFile('output.jpg', (err, info) => { if (err) { console.error('Error converting image format:', err); } else { console.log('Image format converted successfully:', info); } });
Sharp.js supports several formats, including WebP, TIFF, and GIF. You can convert images to any of these formats by using the toFormat
method.
4. Rotating an Image Using Node.js
Rotating images is another useful feature that Sharp.js supports.
sharp('input.png') .rotate(90) // Rotate by 90 degrees .toFile('rotated.png', (err, info) => { if (err) { console.error('Error rotating image:', err); } else { console.log('Image rotated successfully:', info); } });
This will rotate the image by 90 degrees and save it as rotated.png
.
5. Applying Filters Using Node.js
Sharp.js also supports applying filters to images, such as blur, sharpen, or greyscale.
sharp('input.png') .blur(5) // Apply a blur effect with a radius of 5 .toFile('blurred.png', (err, info) => { if (err) { console.error('Error applying blur effect:', err); } else { console.log('Blur effect applied successfully:', info); } });
You can replace blur(5)
with other filter methods like sharpen()
, grayscale()
, or normalize()
to achieve different effects.
Conclusion
Sharp.js is undoubtedly one of the best image processing libraries for Node.js
, offering a fast, efficient, and easy-to-use solution for handling image manipulation tasks.
With its wide range of features, including resizing, cropping, format conversion, and filters, Sharp.js can help you build high-performance applications that need to process images at scale.