Whether you’re building a portfolio website, an e-commerce platform, or a social media app, the need to handle user-uploaded images is a common requirement.
In this comprehensive guide, we’ll explore how to upload an image in Laravel, covering everything from setting up your project to troubleshooting common errors.
By the end of this guide, you’ll know how to use Laravel’s powerful file storage capabilities to integrate image uploads into your projects seamlessly.
So, let’s get started!
Table of Contents
How to Upload Images in Laravel
Follow the steps below to add image upload functionality to your Laravel project.
1. Setting Up Your Laravel Project for Image Upload
Setting up the necessary file storage and configuration is important when working with image uploads in a Laravel application. Laravel provides a robust file storage system that allows you to manage file uploads, including images, easily.
First, you’ll need to configure the file storage driver in your config/filesystems.php
file. Laravel supports several storage drivers, such as local, Amazon S3, and others. For this example, we’ll use the local storage driver, which stores the uploaded files in the storage/app/public
directory.
Next, you’ll need to create a symbolic link between the storage/app/public
directory and the public/storage
directory. This allows the uploaded images to be accessible through the web server. You can create this link by running the php artisan storage:link
command in your terminal.
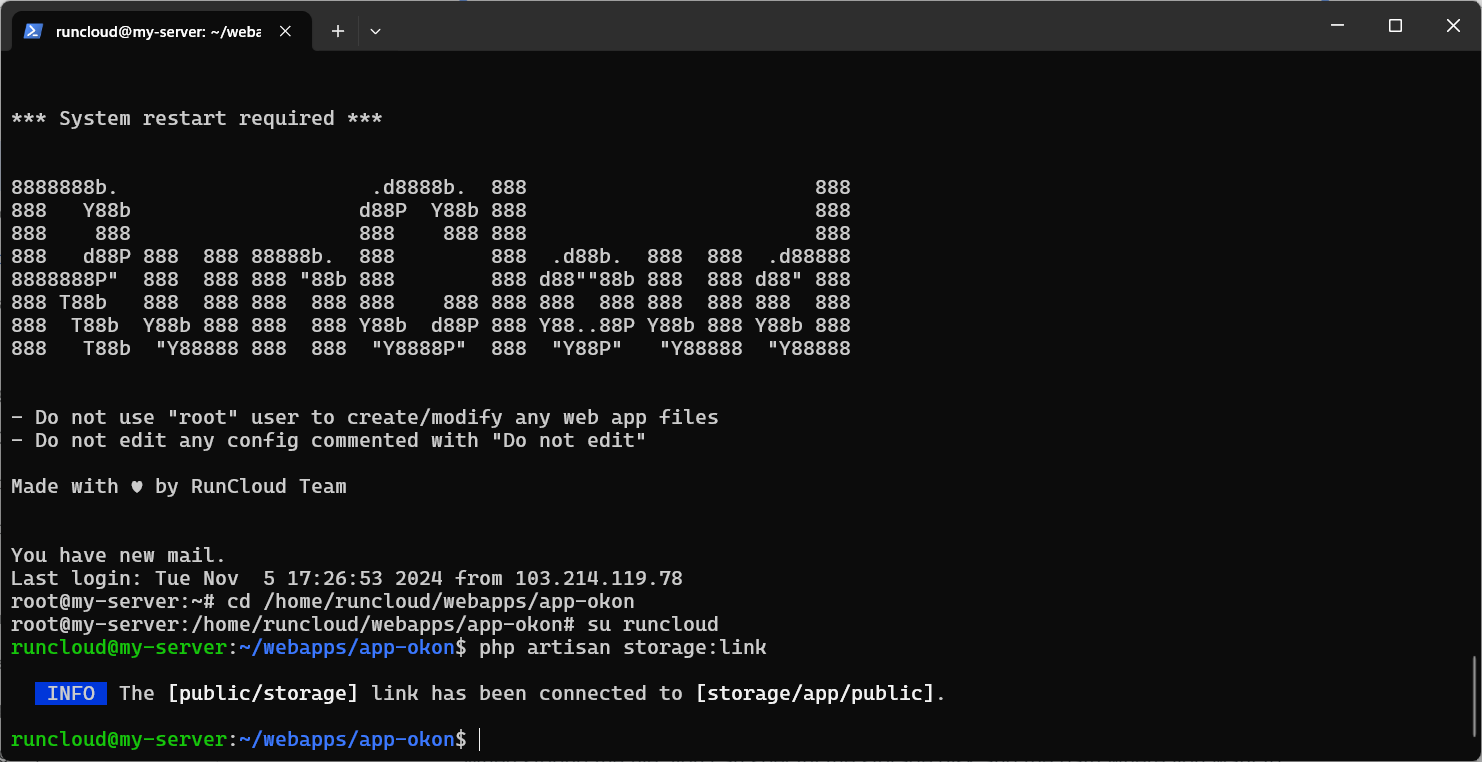
Suggested read: Laravel With Git Deployment The Right Way
2. Creating an Image Upload Form
After creating the symlink, you’ll need to create a form in your Laravel application’s view to allow users to upload images. This form should include an <input> element of type file to allow users to select the image they want to upload.
You’ll also need to include the CSRF token in your form, which helps protect your application from cross-site request forgery attacks. You can do this by using the @csrf directive in your Blade template.
For example, if you want to upload images using the POST method to submit them to the upload.store route, you can use the following code snippet:
<form action="{{ route('upload.store') }}" method="POST" enctype="multipart/form-data">
@csrf
<input type="file" name="image" id="image">
<button type="submit">Upload</button>
</form>
Suggested read: How to Check Laravel Project Version Installed in CMD?
3. Handling Image Upload in Laravel Controller
Once the user submits the image upload form, you’ll need to handle the file in your Laravel controller. You can use the $request->file() method to access the uploaded file and then store it using the $request->file()->store()
method.
When storing the file, you can specify the storage disk and the path where you want to save the file. For example, you could save the file in the public/images directory using the following code:
public function store(Request $request)
{
$request->validate([
'image' => 'required|image|max:2048',
]);
$imagePath = $request->file('image')->store('public/images');
// Save the image path to the database or perform other actions
return redirect()->route('upload.create')->with('success', 'Image uploaded successfully.');
}
This code snippet first validates the incoming request, ensuring that the image field is required, the file is an image, and the file size is no greater than 2MB. It then stores the uploaded image in the public/images directory using the store() method.
After storing the file, you can save the file path in your database so that you can later retrieve and display the uploaded image.
Suggested read: Laravel Octane – What It Is, Why It Matters & Getting Started
4. Displaying Uploaded Images
To display the uploaded images, you can use the asset() helper function in your Blade template. This function will generate the appropriate URL for the file based on your application’s configuration.
For example, if you saved the file in the public/images directory, you can display the image using the following code:
<img src="{{ asset('storage/images/' . $image->filename) }}" alt="{{ $image->filename }}">
Suggested read: Setting Up Local WordPress Dev in Minutes Using Laravel Valet
5. Security Considerations for Image Upload in Laravel
When handling image uploads in your Laravel application, it’s essential to consider security measures to prevent malicious file uploads and other vulnerabilities.
- One important security consideration is file validation. You should always validate the file type, size, and other attributes to ensure that the uploaded file is a valid image and doesn’t exceed your application’s size limits.
- Another important security consideration is file path sanitization. To prevent directory traversal attacks, you should always sanitize the file path before storing or displaying the uploaded images.
- Finally, you should consider implementing additional security measures, such as restricting file types, scanning uploaded files for malware, and limiting the number of uploads per user or per session.
Suggested read: How To Optimize Laravel for Performance (8 Expert Tips)
Troubleshooting Common Errors During Laravel Image Upload
When working with image uploads in a Laravel application, you may encounter various errors and issues. Let’s explore some common errors and how to troubleshoot them.
“File Too Large” Error
One common error that can occur during image uploads is the “File too large” error. This typically happens when the uploaded file exceeds the maximum file size allowed by your server configuration or Laravel application settings.
To troubleshoot this issue, you should first check your php.ini file and ensure that the upload_max_filesize
and post_max_size
directives are set to values that accommodate your expected file sizes. You can also check the config/filesystems.php
file in your Laravel application and update the max_size option for the relevant disk configuration.
If the issue persists, you can try adding a specific file size validation rule in your form request:
$request->validate([
'image' => 'required|image|max:2048',
]);
This will ensure that the uploaded file is no larger than 2MB (2048 kilobytes).
Suggested read: The 10 Best PHP Frameworks (Complete Guide)
“Invalid File Type” Error
Another common error is the “Invalid file type” error, which occurs when the uploaded file is not a valid image format (e.g., JPG, PNG, GIF).
To troubleshoot this issue, you should first check the mimes validation rule in your form request:
$request->validate([
'image' => 'required|image|mimes:jpeg,png,gif',
]);
This rule ensures that the uploaded file is a valid image and that the file extension matches the specified MIME types (in this case, JPEG, PNG, and GIF).
If the issue persists, you can also try using the image rule instead of the mimes rule, as the image rule performs more comprehensive validation and ensures that the uploaded file is a valid image, regardless of the file extension:
$request->validate([
'image' => 'required|image',
]);
File Ownership and Permissions Issues
Sometimes, you may encounter issues with file ownership and permissions when trying to upload images. This can happen if your web server doesn’t have the necessary permissions to write to the storage directory.
To troubleshoot this issue, you should first check the file permissions of the storage/app/public directory (or the directory where you’re storing the uploaded images). Ensure that the web server user (e.g., www-data on Ubuntu, apache on CentOS/RHEL) has write permissions to this directory.
You can also try running the php artisan storage:link
command to create a symbolic link between the storage/app/public
directory and the public/storage
directory, which can help resolve some permission-related issues.
Tip: If you are using RunCloud, you can resolve this issue by going to the “Tools” tab and clicking the “Fix Ownership” button.
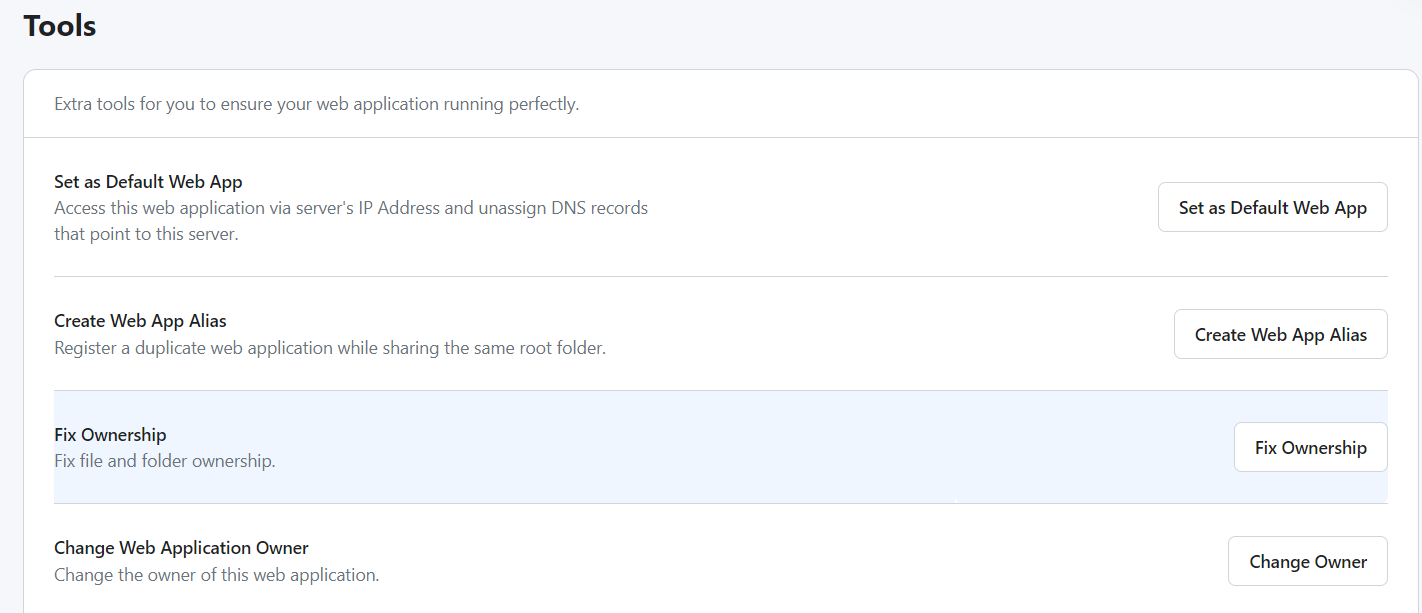
Inconsistent Image Display
If you’re experiencing issues with inconsistently displaying uploaded images, it could be due to caching or a misconfiguration in your application’s routing or asset handling.
To troubleshoot this, you can try adding a timestamp or a unique query parameter to the image URL when displaying the image in your Blade templates:
<img src="{{ asset('storage/images/' . $image->filename . '?v=' . $image->updated_at->timestamp) }}" alt="{{ $image->filename }}">
This will ensure that the browser always fetches the latest version of the image rather than relying on a cached version.
Additionally, you can check your application’s asset configuration in the config/filesystems.php file and ensure that the correct disk and URL are being used for image storage and retrieval.
Suggested read: How To Configure LSCache for Laravel (Configuration Guide)
Wrapping up
In this guide, we’ve explored the ins and outs of image uploads in Laravel. From setting up your project to handle file storage, creating image upload forms, processing uploads in your controllers, and displaying the uploaded images, you should now have a better understanding of the key concepts involved.
However, the journey doesn’t end here. As your Laravel application grows, managing the infrastructure and hosting environment becomes increasingly important – this is where RunCloud comes it.
RunCloud is a powerful and user-friendly platform that simplifies the deployment and management of your Laravel applications.
RunCloud makes building and launching your Laravel applications significantly faster and easier. We handle complex server tasks, like setting up servers, managing SSL certificates, and integrating with popular cloud providers. This means you spend less time on tedious setup and more time building awesome features for your users.
Experience the benefits of simplified Laravel hosting. Start your free trial with RunCloud today!
FAQs on Image Upload in Laravel
How to Display an Image in Laravel
To display an uploaded image in your Laravel application, you can use the asset() helper function to generate the appropriate URL for the image. This function will generate the correct URL based on your application’s configuration, even if the image is stored outside the public directory. For example, if you stored an image in th storage/app/public/images directory, you can display it using the following code:
<img src="{{ asset('storage/images/example.jpg') }}" alt="Example Image">
How to Upload PDF Files using Laravel
Uploading PDF files in Laravel is very similar to uploading images. You can use the same file upload process, but you’ll need to adjust the file validation to accept PDF files instead of images.
For example, you can use the mimes rule in your form request validation to ensure that the uploaded file is a PDF:
$request->validate([
'file' => 'required|mimes:pdf',
]);
After the file is uploaded, you can store the file path in your database and display a download link for the PDF in your application.
How to Put Public Images in Laravel
To make uploaded images publicly accessible in your Laravel application, you can store them in the public directory instead of the storage/app/public directory.
First, create a new directory, such as public/images, in your public folder. Then, update your file upload logic to store the images in this directory:
$imagePath = $request->file('image')->store('images', 'public');
Finally, you can display the images using the asset() helper function, as you would for any other public asset in your application.
How to Add Image Validation in Laravel
To add image validation to your Laravel application, you can use the image rule in your form to request validation. This rule ensures that the uploaded file is a valid image and meets certain criteria, such as file size and MIME type.
Here’s an example of how you can add image validation to your form request:
$request->validate([
'image' => 'required|image|max:2048'
]);
This will ensure that the image field is required, the file is a valid image, and the file size is no larger than 2MB.
You can also use other validation rules, such as mimes or dimensions, to refine your image validation requirements further.
How to Get Image Type in Laravel
To get the type of image uploaded to your Laravel application, you can use the getClientOriginalExtension() method on the UploadedFile object.
For example, in your controller:
$image = $request->file('image');
$imageType = $image->getClientOriginalExtension();
This will give you the file extension of the uploaded image, which you can then use to determine the image type.
Alternatively, you can use the getMimeType() method to get the MIME type of the uploaded image:
$image = $request->file('image');
$imageMimeType = $image->getMimeType();
This can be useful for additional validation or processing of the uploaded image